[Java] JDBC 연습 02개발자가 되기까지 (2023.08.16~2024.04.15)/[Basic] Java2023. 11. 29. 23:34
Table of Contents
<문제>
https://rlog0918.tistory.com/150 에서 했던 JDBC에서 3번 상품검색(상세)를 할 때 마다 readCount가 +1 되고 2번 상품목록과 3번 상품검색(상세)에서 readCount가 보이게 하라 |
<방법>
Product class
package JDBC;
public class Product {
// ...(기존 코드)
// readCount 변수 추가
private int readCount;
// ... (기존 코드)
// 상품리스트 -> pno, pname, price + readCount 추가
public Product(int pno, String pnme, int price, int readCount) {
this.pno = pno;
this.pname = pnme;
this.price = price;
this.readCount = readCount;
}
// 상품상세 -> all + readCount 추가
public Product(int pno, String pnme, int price, String regdate, String madeby, int readCount) {
this.pno = pno;
this.pname = pnme;
this.price = price;
this.regdate = regdate;
this.madeby = madeby;
this.readCount = readCount;
}
// ... (기존코드)
// getter/setter readCount 추가
public int getReadCount() {
return readCount;
}
public void setReadCount(int readCount) {
this.readCount = readCount;
}
// readCount 추가
@Override
public String toString() {
return "Product [pno=" + pno + ", pname=" + pname + ", price=" + price + ", regdate=" + regdate + ", madeby="
+ madeby + ", readCount=" + readCount + "]";
}
}
ProductServiceImpl class
package JDBC;
import java.util.List;
public class ProductServiceImpl implements Service {
// ... (기존코드)
@Override
public Product detail(int pno) {
System.out.println("detail_service success!!");
// readcount 처리
int isOk = dao.readCountUpdate(pno);
return (isOk > 0) ? dao.selectOne(pno) : null;
}
// ... (기존코드)
}
DAO class
package JDBC;
import java.util.List;
public interface DAO {
// ... (기존코드)
int readCountUpdate(int pno);
}
ProductDAOImpl class
package JDBC;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
public class ProductDAOImpl implements DAO {
// ... (기존코드)
@Override
public List<Product> selectAll() {
System.out.println("selectAll DAO Success!!");
query = "select * from product order by pno";
List<Product> list = new ArrayList<>();
try {
pst = conn.prepareStatement(query);
ResultSet rs = pst.executeQuery();
while(rs.next()) {
int pno = rs.getInt("pno");
// pno, pname, price
list.add(new Product(pno,
rs.getString("pname"),
rs.getInt("price"),
// readCount 추가
rs.getInt("readCount")
));
}
return list;
} catch (SQLException e) {
System.out.println("selectAll error");
e.printStackTrace();
}
return null;
}
@Override
public Product selectOne(int pno) {
System.out.println("selectOne DAO Success!!");
query = "select * from product where pno = ?";
try {
pst = conn.prepareStatement(query);
pst.setInt(1, pno);
ResultSet rs = pst.executeQuery();
if(rs.next()) {
return new Product(
rs.getInt("pno"),
rs.getString("pname"),
rs.getInt("price"),
rs.getString("regdate"),
rs.getString("madeby"),
// readCount 추가
rs.getInt("readCount")
);
}
} catch (SQLException e) {
System.out.println("selectOne error");
e.printStackTrace();
}
return null;
}
// ... (기존코드)
// readCount+1 Method
@Override
public int readCountUpdate(int pno) {
System.out.println("readCount DAO Success!!");
query = "update product set readCount = readCount+1 where pno = ?";
try {
pst = conn.prepareStatement(query);
pst.setInt(1, pno);
return pst.executeUpdate();
} catch (SQLException e) {
System.out.println("readCount error");
e.printStackTrace();
}
return 0;
}
}
[Java] JDBC 연습 02
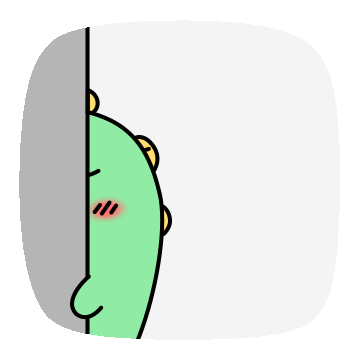
(다음 게시물 예고편)
[Java] Eclipse JSTL / JSP 개발환경 설정하기
728x90
@rlozlr :: 얼렁뚱땅 개발자
얼렁뚱땅 주니어 개발자
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!