[Java] JDBC 연습 01개발자가 되기까지 (2023.08.16~2024.04.15)/[Basic] Java2023. 11. 29. 19:55
Table of Contents
<문제>
<조건> Menu List 1. 상품등록 => pname, price, madeby 2. 상품목록 => pno, pname, price 3. 상품검색(상세) => all 4. 상품수정 => pno, pname, price, madeby 5. 상품삭제 6. 종료 |
<방법>
sql
/*
product 테이블 생성
pno : 제품등록번호 // ai, 기본키
pname : 이름 varchar(200)
price : 가격 int
regdate : 등록일자 datetime default now()
madeby : 설명
*/
CREATE TABLE product(
pno INT NOT NULL AUTO_INCREMENT,
pname VARCHAR(200) NOT NULL,
price INT NOT NULL DEFAULT 0,
regdate DATETIME DEFAULT NOW(),
madeby TEXT,
PRIMARY KEY(pno));
DatabaseConnection class
package JDBC;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
// DB 접속
// DB Driver, URL, user, password
// driver : com.mysql.cj.jdbc.Driver
// url : jdbc:mysql://localhost:3306/db명
// user :
// password :
// 싱글톤 패턴을 사용하여 단 하나의 DatabaseConnection 객체를 생성하도록 함
private static DatabaseConnection dbc = new DatabaseConnection();
// DB 연결 객체
private Connection conn = null;
// JDBC 드라이버 클래스명
private String jdbcDriver = "com.mysql.cj.jdbc.Driver";
// DB URL
private String jdbcUrl = "jdbc:mysql://localhost:3306/db명";
// 생성자에서 DB 접속 수행
private DatabaseConnection() {
// 반드시 try-catch 블록으로 예외 처리
try {
// JDBC 드라이버 클래스 로드
Class.forName(jdbcDriver);
// DriverManager를 사용하여 DB 연결
conn = DriverManager.getConnection(jdbcUrl, "권한을 가진id", "권한을 가진 id의 pwd");
} catch (ClassNotFoundException e) {
System.out.println("드라이버를 찾을 수 없음");
e.printStackTrace();
} catch (SQLException e) {
System.out.println("연결정보가 정확하지 않음");
e.printStackTrace();
}
}
// 싱글톤 패턴에서 단일 객체를 반환하는 메서드
static DatabaseConnection getInstance() {
return dbc;
}
// 데이터베이스 연결 객체를 반환하는 메서드
Connection getConnection() {
return conn;
}
}
Product class
package JDBC;
public class Product {
private int pno;
private String pname;
private int price;
private String regdate;
private String madeby;
public Product() {}
// 상품등록 -> pname, price, madeby
public Product(String pnme, int price, String madeby) {
this.pname = pnme;
this.price = price;
this.madeby = madeby;
}
// 상품리스트 -> pno, pname, price
public Product(int pno, String pnme, int price) {
this.pno = pno;
this.pname = pnme;
this.price = price;
}
// 상품상세 -> all
public Product(int pno, String pnme, int price, String regdate, String madeby) {
this.pno = pno;
this.pname = pnme;
this.price = price;
this.regdate = regdate;
this.madeby = madeby;
}
// 상품수정 -> pno, pname, price, madeby
public Product(int pno, String pnme, int price, String madeby) {
this.pno = pno;
this.pname = pnme;
this.price = price;
this.madeby = madeby;
}
public int getPno() {
return pno;
}
public void setPno(int pno) {
this.pno = pno;
}
public String getPname() {
return pname;
}
public void setPname(String pname) {
this.pname = pname;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public String getRegdate() {
return regdate;
}
public void setRegdate(String regdate) {
this.regdate = regdate;
}
public String getMadeby() {
return madeby;
}
public void setMadeby(String madeby) {
this.madeby = madeby;
}
@Override
public String toString() {
return "Product [pno=" + pno + ", pname=" + pname + ", price=" + price + ", regdate=" + regdate + ", madeby="
+ madeby + "]";
}
}
ProductMain class
package JDBC;
public class ProductMain {
public static void main(String[] args) {
new ProductController();
}
}
ProductController class
package JDBC;
import java.util.List;
import java.util.Scanner;
public class ProductController {
/* 상품등록, 상품리스트보기, 상품상세보기, 상품수정, 상품삭제
* Controller <-> Service <-> DAO <-> DB
* Controller에서 분기처리 모든 메뉴를 처리 */
private Scanner scan;
private Service svc; // package에 inteface로 생성
private Boolean flag; // 종료변수
public ProductController() {
scan = new Scanner(System.in);
svc = new ProductServiceImpl(); // service 구현체 class로 생성
flag = true;
printMenu();
}
private void printMenu() {
// flag가 true면 계속 메뉴출력
while(flag) {
System.out.println("--상품관리프로그램--");
System.out.println("1.상품등록 | 2. 상품목록 | 3. 상품검색(상세)");
System.out.println("4.상품수정 | 5. 상품삭제 | 6. 종료");
System.out.print(">> ");
int menu = scan.nextInt();
switch(menu) {
case 1: register(); break;
case 2: list(); break;
case 3: detail(); break;
case 4: modify(); break;
case 5: remove(); break;
default : flag = false; break;
}
}
}
private void remove() {
System.out.print("삭제할 상품번호 >> ");
int pno = scan.nextInt();
int isOk = svc.remove(pno);
System.out.println(" >>> 상품삭제 " + ((isOk > 0) ? "OK" : "Fail"));
}
private void modify() {
// 상품번호를 받아서 해당 상품의 내용을 수정 => 번호, 이름, 가격, 설명 수정
// update 구문 사용
System.out.print("수정할 상품번호 >> ");
int pno = scan.nextInt();
System.out.print("상품명 >> ");
scan.nextLine();
String name = scan.nextLine();
System.out.print("가격 >> ");
int price = scan.nextInt();
System.out.print("상품상세내역 >> ");
scan.nextLine();
String madeby = scan.nextLine();
Product p = new Product(pno, name, price, madeby);
// update 구문사용 => 리턴은 1 또는 0
int isOk = svc.modify(p);
System.out.println(" >>> 상품수정 " + ((isOk > 0) ? "OK" : "Fail"));
}
private void detail() {
System.out.print("상품번호 >> ");
int pno = scan.nextInt();
Product p = svc.detail(pno);
System.out.println(p);
}
// 상품목록
private void list() {
List<Product> list = svc.list();
for(Product p : list) {
System.out.println(p);
}
}
// 상품등록
private void register() {
System.out.print("상품명 >> ");
scan.nextLine();
String name = scan.nextLine();
System.out.print("가격 >> ");
int price = scan.nextInt();
System.out.print("상품상세내역 >> ");
scan.nextLine();
String madeby = scan.nextLine();
Product p = new Product(name, price, madeby);
// svc에게 등록을 요청하는 메서드를 작성
// insert를 하게되면 리턴되는 값은 몇개의 행이 insert되었는지 값이 리턴
// isOk insert 후 리턴되는 값을 저장 잘되면 1이 리턴, 안되면 0이 리턴
int isOk = svc.register(p);
System.out.println(" >>> 상품등록 " + ((isOk > 0) ? "OK" : "Fail"));
}
}
Service class
package JDBC;
import java.util.List;
public interface Service {
int register(Product p);
List<Product> list();
Product detail(int pno);
int modify(Product p);
int remove(int pno);
}
ServiceImpl class
package JDBC;
import java.util.List;
public class ProductServiceImpl implements Service {
// ServiceImpl <=> DAO
private DAO dao; // interface로 생성 => DAOImpl로 구현
public ProductServiceImpl() {
dao = new ProductDAOImpl(); // 구현체 class로 생성
}
@Override
public int register(Product p) {
System.out.println("register_service success!!");
// dao에 사용되는 메서드는 실제 DB에서 사용되는 명령어와 비슷하게 명령어 작성
return dao.insert(p);
}
@Override
public List<Product> list() {
System.out.println("list_service success!!");
return dao.selectAll();
}
@Override
public Product detail(int pno) {
System.out.println("detail_service success!!");
return dao.selectOne(pno);
}
@Override
public int modify(Product p) {
System.out.println("modify_service success!!");
return dao.update(p);
}
@Override
public int remove(int pno) {
System.out.println("remove_service success!!");
return dao.delete(pno);
}
}
DAO class
package JDBC;
import java.util.List;
public interface DAO {
int insert(Product p);
List<Product> selectAll();
Product selectOne(int pno);
int update(Product p);
int delete(int pno);
}
ProductDAOImpl class
package JDBC;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
public class ProductDAOImpl implements DAO {
// DB와 연결
// DB와 실제 연결하는 구문
// DB와 연결하는 역할
private Connection conn;
// SQL구문을 실행시키는 기능을 갖는 객체
private PreparedStatement pst;
// query문 저장
private String query="";
public ProductDAOImpl() {
// DB 연결정보를 설정하는 class 싱글톤으로 생성
DatabaseConnection dbc = DatabaseConnection.getInstance();
conn = dbc.getConnection();
}
// SQL 구문 처리
// executeQuery() / select 구문에서 사용 ResultSet이 리턴
// executeUpdate() / insert, update, delete 별도의 리턴이 없고, 0 / 1로만 리턴
@Override
public int insert(Product p) {
System.out.println("insert DAO Success!!");
query = "insert into product(pname, price, madeby) values(?,?,?)";
try {
pst = conn.prepareStatement(query);
// ?값 setting
pst.setString(1, p.getPname());
pst.setInt(2, p.getPrice());
pst.setString(3, p.getMadeby());
return pst.executeUpdate();
} catch (Exception e) {
System.out.println("insert error!!");
e.printStackTrace();
}
return 0;
}
@Override
public List<Product> selectAll() {
System.out.println("selectAll DAO Success!!");
query = "select * from product order by pno";
List<Product> list = new ArrayList<>();
try {
pst = conn.prepareStatement(query);
ResultSet rs = pst.executeQuery();
while(rs.next()) {
int pno = rs.getInt("pno");
// pno, pname, price
list.add(new Product(pno,
rs.getString("pname"),
rs.getInt("price")));
}
return list;
} catch (SQLException e) {
System.out.println("selectAll error");
e.printStackTrace();
}
return null;
}
@Override
public Product selectOne(int pno) {
System.out.println("selectOne DAO Success!!");
query = "select * from product where pno = ?";
try {
pst = conn.prepareStatement(query);
pst.setInt(1, pno);
ResultSet rs = pst.executeQuery();
if(rs.next()) {
return new Product(
rs.getInt("pno"),
rs.getString("pname"),
rs.getInt("price"),
rs.getString("regdate"),
rs.getString("madeby")
);
}
} catch (SQLException e) {
System.out.println("selectOne error");
e.printStackTrace();
}
return null;
}
@Override
public int update(Product p) {
System.out.println("update DAO Success!!");
query = "update product set pname=?, price=?, regdate=now(), madeby=? where pno=?";
try {
pst = conn.prepareStatement(query);
pst.setString(1, p.getPname());
pst.setInt(2, p.getPrice());
pst.setString(3, p.getMadeby());
pst.setInt(4, p.getPno());
return pst.executeUpdate();
} catch (SQLException e) {
System.out.println("update error");
e.printStackTrace();
}
return 0;
}
@Override
public int delete(int pno) {
System.out.println("delete DAO Success!!");
query = "delete from product where pno=?";
try {
pst = conn.prepareStatement(query);
pst.setInt(1, pno);
return pst.executeUpdate();
} catch (SQLException e) {
System.out.println("delete error");
e.printStackTrace();
}
return 0;
}
}
[Java] JDBC 연습 01
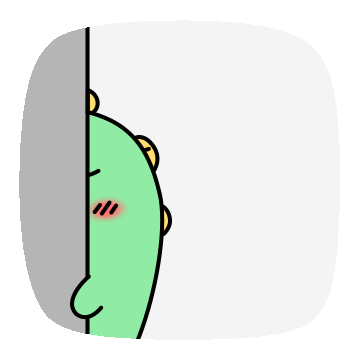
(다음 게시물 예고편)
[Java] JDBC 연습 02
728x90
@rlozlr :: 얼렁뚱땅 개발자
얼렁뚱땅 주니어 개발자
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!