[Java] 학생성적 Stream을 활용하여 출력개발자가 되기까지 (2023.08.16~2024.04.15)/[Basic] Java2023. 10. 15. 18:47
Table of Contents
public class Student {
// 이름과 점수만 가지고 있는 클래스 생성
// 멤버변수와, 생성자, getter/setter, toString
private String name;
private int score;
public Student() { }
public Student(String name, int score) {
this.name = name;
this.score = score;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
@Override
public String toString() {
return name + " : " + score;
}
}
import java.util.ArrayList;
public class GradeCard {
public static void main(String[] args) {
// Student 클래스를 가지는 List 생성
// 5명 정도의 값을 추가
ArrayList<Student> std = new ArrayList<>();
std.add(new Student("아이언맨",90));
std.add(new Student("헐크",76));
std.add(new Student("스파이더맨",48));
std.add(new Student("스트레인지",89));
std.add(new Student("슈퍼맨",45));
// stream 구성 후 출력
// toString 존재할 경우
std.stream().forEach(System.out::println);
System.out.println("---------------");
// toString이 없을 경우
// {} 처리 구문이 많을 경우
std.stream().forEach(n ->{
String name = n.getName();
int score = n.getScore();
System.out.println(name+" : "+score);
});
System.out.println("---------------");
// 성적 합계 출력
int scoreSum = std.stream()
.mapToInt(t->t.getScore())
.sum();
System.out.println("성적합계: "+scoreSum);
// 점수가 70점 이상인 인원수 출력
Long count = std.stream()
.mapToInt(t->t.getScore())
.filter(n-> n>=70)
.count();
System.out.println("70점 이상: "+count+"명");
}
}
[Java] 학생성적 Stream을 활용하여 출력 끝!
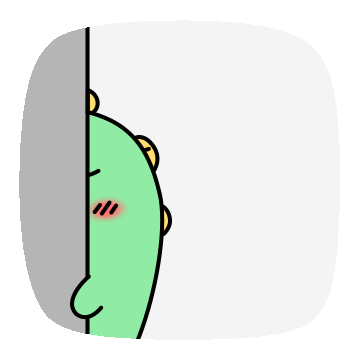
(다음 게시물 예고편)
[Java] List와 Map을 활용하여 학생 관리프로그램 만들기
728x90
@rlozlr :: 얼렁뚱땅 개발자
얼렁뚱땅 주니어 개발자
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!