[Java] Customer Class의 상속을 받아 고객 관리프로그램 만들기개발자가 되기까지 (2023.08.16~2024.04.15)/[Basic] Java2023. 10. 8. 04:43
Table of Contents
<문제>
Custoemr Class의 상속을 받아 고객 관리프로그램 만들기 |
고객 클래스 - 일반고객 / Gold 고객 / VIP 고객 - 멤버변수 - 고객ID : int customerID - 고객이름 : String custoemrName - 고객등급 : String custoemrGrade - 보너스포인트 : int bonusPoint - 보너스포인트 적립비율 : double bonusRatio ======================================================================= Customer Class - 기본 customerGrade = Silver - 기본 dobule bonusRatio = 0.01 - Method - 보너스 적립 계산 ( calcPrice(int price) ) -> 보너스를 적립하고, 할인여부 체크해서 구매 price 리턴 구매 금액을 주고, 적립 보너스 계산, bonusPoint 누적, 실제 구매금액 리턴 - 출력 ( customerInfo() ) -> 홍길동님의 등급은 VIP이며, 보너스 포인트는 1000점입니다. 전담 상담사 번호는 1111입니다, (VIP만 출력) ======================================================================= <조건> - Silver 등급 : 할인 없음 / 보너스 포인트 1% 적립 - Gold 등급 : 10% 할인 / 보너스 포인트 2% 적립 - VIP 등급 : 20% 할인 / 보너스 포인트 5% 적립 / 전담 상담사 ( int agentID ) |
<방법>
public class Customer {
protected int customerID;
protected String customerName;
protected int bonusPoint;
protected String customerGrade = "Silver";
protected double bonusRatio = 0.01;
protected double saleRatio = 1;
public Customer () { }
public Customer(String customerName, int bonusPoint) {
this.customerName = customerName;
this.bonusPoint = bonusPoint;
}
// 보너스 적립 게산 메서드(메서드명 : calcPirce(int price))
// 보너스를 적립하고, 할인여부 체크하여 구매 price 리턴
public void calcPirce(int price) {
int insertBonus = (int) (price*bonusRatio);
bonusPoint += insertBonus;
System.out.println("적립된 보너스> "+ insertBonus+"점");
System.out.println("누적된 보너스> "+ bonusPoint+"점");
System.out.println("원가> "+ price+"원");
System.out.println("할인금액> "+ (int)(price-(price*saleRatio))+"원");
price = (int)(price*saleRatio);
System.out.println("할인이 적용된 금액> "+ price+"원");
}
// 출력 메서드 (메서드명 : customerInfo())
// 홍길동님의 등급은 VIP이며, 보너스 포인트는 1000점입니다.
// 전담 상담사 번호는 1111입니다. - VIP 클래스에서 추가해주기
public void customerInfo() {
System.out.println("["+customerName+"]님의 등급은 "+ customerGrade +"이며, 보너스 포인트는 "+bonusPoint+"점 입니다.");
}
}
public class GoldCustomer extends Customer{
protected String goldCustomerGrade = "Gold";
protected double goldBonusRatio = 0.02;
protected double goldSaleRatio = 0.9;
public GoldCustomer() {
customerGrade = goldCustomerGrade;
bonusRatio = goldBonusRatio;
saleRatio = goldSaleRatio;
}
public GoldCustomer(String customerName, int bonusPoint) {
super(customerName, bonusPoint);
customerGrade = goldCustomerGrade;
bonusRatio = goldBonusRatio;
saleRatio = goldSaleRatio;
}
@Override
public void calcPirce(int price) {
super.calcPirce(price);
}
@Override
public void customerInfo() {
// TODO Auto-generated method stub
super.customerInfo();
}
}
public class VIPCustomer extends Customer {
//전담상담사를 갖는다. (int agentID)
private int agentID;
protected String vipCustomerGrade = "VIP";
protected double vipBonusRatio = 0.05;
protected double vipSaleRatio = 0.8;
public VIPCustomer() {
customerGrade = vipCustomerGrade;
bonusRatio = vipBonusRatio;
saleRatio = vipSaleRatio;
}
public VIPCustomer(String customerName, int bonusPoint) {
super(customerName, bonusPoint);
customerGrade = vipCustomerGrade;
bonusRatio = vipBonusRatio;
saleRatio = vipSaleRatio;
}
@Override
public void calcPirce(int price) {
super.calcPirce(price);
}
// 전담 상담사 번호는 1111입니다. - VIP 클래스에서 추가해주기
@Override
public void customerInfo() {
super.customerInfo();
agent();
System.out.println("전담 상담사 번호는 "+agentID+"입니다.");
}
//전담사 번호 만들어주기
public int agent() {
agentID = (int)(Math.random()*5000)+1000;
return agentID;
}
}
public class CustomerMain {
public static void main(String[] args) {
Customer c1 = new Customer("스파이더맨", 1000);
GoldCustomer c2 = new GoldCustomer("토르", 3000);
VIPCustomer c3 = new VIPCustomer("아이언맨", 5000);
System.out.println("-------");
int price = 10000;
c1.calcPirce(price);
c1.customerInfo();
System.out.println("-----------");
c2.calcPirce(price);
c2.customerInfo();
System.out.println("-----------");
c3.calcPirce(price);
c3.customerInfo();
}
}
[Java] Customer Class의 상속을 받아 고객 관리프로그램 만들기 끝!
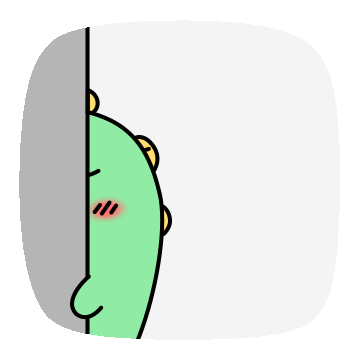
(다음 게시물 예고편)
[Java] 예외처리
728x90
@rlozlr :: 얼렁뚱땅 개발자
얼렁뚱땅 주니어 개발자
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!