[Java] 수강 관리 프로그램 만들기개발자가 되기까지 (2023.08.16~2024.04.15)/[Basic] Java2023. 9. 27. 00:10
Table of Contents
반응형
<문제>
수강 관리 프로그램 만들기 |
ComputerCourse Class 학생의 기본정보 : 이름, 생년월일, 나이, 전화번호 학원 정보 : 학원이름 = "Academy", 지점 수강정보 : 수강과목, 기간 (n개월) 기능 : 학생정보출력, 학원정보출력, 수강정보출력, 학생의 수강정보를 추가 ComputerCourseMain Class 1. ComputerCourse의 객체 생성 후 출력 2. 학생클래스를 담을 수 있는 배열[7]을 생성 후, 7명의 학생을 등록 3. 학생 이름으로 검색 => 모든정보 출력 4. 지점정보로 검색 => 학생정보만 출력 5. 과목을 수강하는 학생만 검색 => 학생정보, 수강정보 출력 |
<방법>
public class ComputerCourse {
// 멤버변수 선언
private String name;
private String birth;
private int age;
private String phone;
// final = 변경불가능한
public final static String company = "Academy";
private String branch;
private String[] course = new String[5]; // 수강과목 5개
private String[] period = new String[5]; // 수강기간 5개
private int cnt; // 배열의 index 처리
// 생성자
public ComputerCourse() {}
public ComputerCourse(String name, String phone, String branch) {
this.name=name;
this.phone=phone;
this.branch=branch;
}
public ComputerCourse(String name, String birth, int age, String phone, String branch) {
this.name=name;
this.birth=birth;
this.age=age;
this.phone=phone;
this.branch=branch;
}
// 학생정보출력 메서드
public void printInfo() {
System.out.println(name+", "+birth+", "+age+", "+phone);
}
// 학원정보출력 메서드
public void printCompany() {
System.out.println(company+" ("+branch+")");
}
// 수강정보출력 메서드
public void printCourse() {
if(course.length == 0 || cnt ==0) {
System.out.println("수강 이력이 없습니다.");
return; // 출력을 멈추고 메서드 끝내기
}
for(int i =0; i<cnt; i++) {
System.out.print(course[i]+"("+period[i]+") ");
}
System.out.println();
}
// 학생의 수강정보 추가 메서드
public void insertCourse(String course, String period) {
this.course[cnt] = course;
this.period[cnt] = period;
cnt++; // index 증가
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getBirth() {
return birth;
}
public void setBirth(String birth) {
this.birth = birth;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getBranch() {
return branch;
}
public void setBranch(String branch) {
this.branch = branch;
}
public String[] getCourse() {
return course;
}
public void setCourse(String[] course) {
this.course = course;
}
public String[] getPeriod() {
return period;
}
public void setPeriod(String[] period) {
this.period = period;
}
public int getCnt() {
return cnt;
}
public static String getCompany() {
return company;
}
}
import java.util.Scanner;
public class ComputerCourseMain {
public static void main(String[] args) {
// ComputerCourse의 객체 생성 후 출력
ComputerCourse std1 = new ComputerCourse("야도란","010-1111-1111","서울점") ;
std1.printInfo();
std1.printCompany();
std1.insertCourse("java", "6개월");
std1.printCourse();
System.out.println("-------------");
/* 학생클래스를 담을 수 있는 배열[7]을 생성 후
* 7명의 학생을 등록
* */
ComputerCourse[] std = new ComputerCourse[7];
std[0] = std1;
std[1] = new ComputerCourse("피카츄","990101",24,"1111","서울");
std[2] = new ComputerCourse("꼬부기","990112",25,"2222","경기");
std[3] = new ComputerCourse("토게피","990123",26,"3333","인천");
std[4] = new ComputerCourse("파이리","990104",27,"4444","서울");
std[5] = new ComputerCourse("망나뇽","990204",21,"5555","서울");
std[6] = new ComputerCourse("롱스톤","990304",23,"6666","인천");
std[0].insertCourse("DB", "1개월");
std[1].insertCourse("html", "2개월");
std[1].insertCourse("java", "6개월");
// 학생이름으로 검색 => 모든정보 출력
Scanner scan = new Scanner(System.in);
int cnt =0;
System.out.print("학생명> ");
String searchName = scan.next();
System.out.println(searchName+" 님의 정보----------");
for(int i =0; i<std.length; i++) {
if(std[i].getName().equals(searchName)) {
std[i].printInfo();
std[i].printCompany();
std[i].printCourse();
cnt++;
}
}
if(cnt == 0) {
System.out.println("존재하지 않는 학생입니다.");
}
System.out.println("--------------------------");
System.out.println();
// 과목을 수강하는 학생만 검색 => 학생정보, 수강정보 출력
System.out.print("지점명> ");
String searchBranch = scan.next();
System.out.println(searchBranch +"지점의 학생들 정보--------");
for(ComputerCourse str : std) {
if(str.getBranch().equals(searchBranch)) {
str.printInfo();
}
}
System.out.println("--------------------------");
System.out.println();
// 지점정보로 검색 => 학생정보만 출력
System.out.print("과목명> ");
String searchCourse = scan.next();
System.out.println(searchCourse +"를 수강하는 학생들 정보--------");
for(ComputerCourse str : std) {
for(int i = 0; i <str.getCnt(); i++) {
if(str.getCourse()[i].equals(searchCourse)) {
System.out.println("==========================");
str.printInfo();
str.printCourse();
}
}
}
System.out.println("--------------------------");
System.out.println();
}
}
[Java] 수강 관리 프로그램 만들기 끝!
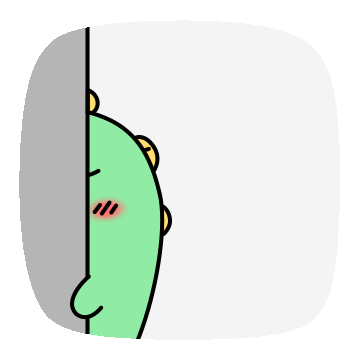
(다음 게시물 예고편)
[Java] 상품 등록 Class 만들기
728x90
반응형
@rlozlr :: 얼렁뚱땅 개발자
얼렁뚱땅 주니어 개발자
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!