![[Spring] 11. 게시물 상세 / 수정 / 삭제](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FbnYxC9%2FbtsDBAuOi41%2FKk86SUKpa88hSUU5fz9491%2Fimg.png)
detail.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<jsp:include page="../layout/header.jsp"></jsp:include>
<jsp:include page="../layout/nav.jsp"></jsp:include>
<div class="container-md">
<div class="mb-3">
<label for="bno" class="form-label">No.</label>
<input type="text" name="bno" class="form-control" id="bno" readonly value="${bvo.bno }">
</div>
<div class="mb-3">
<label for="title" class="form-label">제목</label>
<input type="text" name="title" class="form-control" id="title" readonly value="${bvo.title }">
</div>
<div class="mb-3">
<label for="writer" class="form-label">작성자</label>
<input type="text" name="writer" class="form-control" id="writer" readonly value="${bvo.writer }">
</div>
<div class="mb-3">
<label for="reg_date" class="form-label">작성일</label>
<span class="badge text-bg-primary">${bvo.readCount }</span>
<input type="text" name="reg_date" class="form-control" id="reg_date" readonly value="${bvo.regAt }">
</div>
<div class="mb-3">
<label for="mod_date" class="form-label">수정일</label>
<input type="text" name="mod_date" class="form-control" id="mod_date" readonly value="${bvo.modAt }">
</div>
<div class="mb-3">
<label for="content" class="form-label">내용</label>
<input type="text" name="content" class="form-control" id="content" readonly value="${bvo.content }">
</div>
<a href="/board/modify?bno=${bvo.bno }"><button type="submit" class="btn btn-success">수정</button></a>
<a href="/board/remove?bno=${bvo.bno }"><button type="button" class="btn btn-danger">삭제</button></a>
<a href="/board/list"><button type="submit" class="btn btn-primary">목록</button></a><br>
</div>
<jsp:include page="../layout/footer.jsp"></jsp:include>
modify.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<jsp:include page="../layout/header.jsp"></jsp:include>
<jsp:include page="../layout/nav.jsp"></jsp:include>
<div class="container-md">
<h3>게시글 수정</h3>
<hr>
<form action="/board/modify" method="post">
<div class="mb-3">
<label for="bno" class="form-label">No.</label>
<input type="text" name="bno" class="form-control" id="bno" readonly value="${bvo.bno }">
</div>
<div class="mb-3">
<label for="title" class="form-label">제목</label>
<input type="text" name="title" class="form-control" id="title" value="${bvo.title }">
</div>
<div class="mb-3">
<label for="writer" class="form-label">작성자</label>
<input type="text" name="writer" class="form-control" id="writer" readonly value="${bvo.writer }">
</div>
<div class="mb-3">
<label for="reg_date" class="form-label">작성일</label>
<input type="text" name="reg_date" class="form-control" id="reg_date" readonly value="${bvo.regAt }">
</div>
<div class="mb-3">
<label for="mod_date" class="form-label">수정일</label>
<input type="text" name="mod_date" class="form-control" id="mod_date" readonly value="${bvo.modAt }">
</div>
<div class="mb-3">
<label for="content" class="form-label">내용</label>
<input type="text" name="content" class="form-control" id="content" value="${bvo.content }">
</div>
<a href="/board/modify?bno=${bvo.bno }"><button type="submit" class="btn btn-success">수정</button></a>
<a href="/board/remove?bno=${bvo.bno }"><button type="button" class="btn btn-danger">삭제</button></a>
<a href="/board/list"><button type="submit" class="btn btn-primary">목록</button></a><br>
</form>
</div>
<jsp:include page="../layout/footer.jsp"></jsp:include>
수정 페이지에서는 조회수를 볼 필요가 없다고 생각해서 조회수는 빼주었다.
BoardController.java
package com.basicWeb.www.controller;
import java.util.List;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import com.basicWeb.www.domain.BoardVO;
import com.basicWeb.www.service.BoardService;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@RequestMapping("/board/*")
@RequiredArgsConstructor
@Controller
public class BoardController {
private final BoardService bsv;
// ... (기존 코드)
@GetMapping({"/detail", "/modify"})
public void detail (Model m, @RequestParam("bno") long bno) {
m.addAttribute("bvo", bsv.getDetail(bno));
}
@PostMapping("/modify")
public String modify (BoardVO bvo, RedirectAttributes re) {
bsv.modify(bvo);
re.addAttribute("bno", bvo.getBno());
return "redirect:/board/detail?bno=" +bvo.getBno();
}
@GetMapping("/remove")
public String remove (BoardVO bvo) {
bsv.remove(bvo);
return "redirect:/board/list";
}
}
controller에서 상세 페이지와 수정 페이지로 이동하는 코드
@GetMapping({"/detail", "/modify"})
public void detail (Model m, @RequestParam("bno") long bno) {
m.addAttribute("bvo", bsv.getDetail(bno));
}
Model은 주로 Controller에서 view로 전달할 데이터를 설정하는 데 사용
@RequestParam은 HTTP 요청의 파라미터를 메서드의 매개변수로 바인딩하는 데 사용
controller에서 게시물을 수정하는 코드
@PostMapping("/modify")
public String modify (BoardVO bvo, RedirectAttributes re) {
bsv.modify(bvo);
re.addAttribute("bno", bvo.getBno());
return "redirect:/board/detail?bno=" +bvo.getBno();
}
RedirectAttributes 주로 리다이렉트할 때 데이터를 유지하고자 할 때 사용
일회성이므로 한 번만 읽을 수 있으며 사용자에게 정보를 주고자 할 때 활용
controller에서 게시물을 삭제하는 코드
@GetMapping("/remove")
public String remove (BoardVO bvo) {
bsv.remove(bvo);
return "redirect:/board/list";
}
BoardService.interface
package com.basicWeb.www.service;
import java.util.List;
import com.basicWeb.www.domain.BoardVO;
public interface BoardService {
void register(BoardVO bvo);
List<BoardVO> getList();
BoardVO getDetail(long bno);
void modify(BoardVO bvo);
void remove(BoardVO bvo);
}
BoardServiceImpl.java
package com.basicWeb.www.service;
import java.util.List;
import org.springframework.stereotype.Service;
import com.basicWeb.www.domain.BoardVO;
import com.basicWeb.www.repository.BoardDAO;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@RequiredArgsConstructor
@Service
public class BoardServiceImpl implements BoardService{
private final BoardDAO bdao;
// ... (기존 코드)
@Override
public BoardVO getDetail(long bno) {
bdao.upReadCount(bno, 1);
return bdao.getDetail(bno);
}
@Override
public void modify(BoardVO bvo) {
bdao.upReadCount(bvo.getBno(), -2);
bdao.update(bvo);
}
@Override
public void remove(BoardVO bvo) {
bdao.delete(bvo);
}
}
상세 페이지에 들어갔을 때 조회수가 +1 되어야 한다.
bdao.upReadCount(bno, 1);
그러나 이렇게 설정할 경우, Controller에서 detail과 modify가 같은 GetMapping을 사용하므로
상세 페이지 들어갈 때 +1, 수정 페이지 들어갈 때 +1, 수정 후 다시 상세페이지에 들어갔을 때 +1이 되므로
수정할 때 조회수를 -2로 설정한다.
bdao.upReadCount(bvo.getBno(), -2);
BoardDAO.interface
package com.basicWeb.www.repository;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import com.basicWeb.www.domain.BoardVO;
public interface BoardDAO {
void register(BoardVO bvo);
List<BoardVO> getList();
BoardVO getDetail(long bno);
void update(BoardVO bvo);
void delete(BoardVO bvo);
void upReadCount(@Param("bno") long bno, @Param("count") int count);
}
@Param을 사용하는 이유는,
SQL이 매개변수를 2개 이상 읽을 수 없기 때문에 Param 어노테이션을 사용하여
매개변수에 이름을 부여해주는 것이다.
boardMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.basicWeb.www.repository.BoardDAO">
<!-- ... (기존 코드) -->
<select id="getDetail" resultType="com.basicWeb.www.domain.BoardVO">
SELECT * FROM board WHERE bno = #{bno}
</select>
<update id="update">
UPDATE board SET
title = #{title},
content = #{content},
mod_at = now()
WHERE bno = #{bno}
</update>
<update id="upReadCount">
UPDATE board SET
read_count = read_count + #{count}
WHERE bno = #{bno}
</update>
<delete id="delete">
DELETE FROM board WHERE bno = #{bno}
</delete>
</mapper>
<상세 페이지>
<수정 페이지>
[Spring] 11. 게시물 상세 / 수정 / 삭제
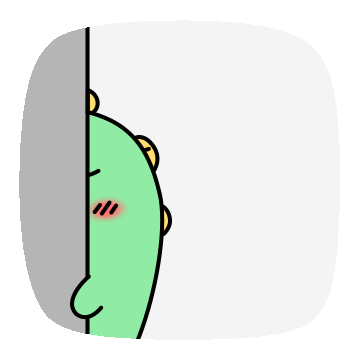
(다음 게시물 예고편)
[Spring] 12. 게시판 - 리스트 페이지네이션
얼렁뚱땅 주니어 개발자
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!